Secure Vibe Coding Guide
Published 04/09/2025
Written by Ken Huang, CSA Fellow, Co-Chair of CSA AI Safety Working Groups.
1: Introduction
Vibe coding is an emerging AI-assisted programming approach where users describe their software requirements in natural language, and a large language model (LLM) generates the corresponding code. This method shifts the programmer's role from manually writing code to guiding, testing, and refining the AI's output. The term was popularized as a casual and experimental way to create software, particularly for quick or informal projects.
1.1 Key Features of Vibe Coding
- Natural Language Input: Users express their ideas or problems conversationally, often using plain language or voice commands instead of traditional programming syntax.
- AI-Generated Code: Large language models handle the coding process by autonomously generating functional code based on user prompts.
- Iterative Refinement: Users refine the generated code by providing feedback to the AI, describing issues or requesting changes until the desired functionality is achieved.
- Minimal Code Understanding: Unlike traditional programming, vibe coding often involves accepting code without fully understanding its implementation. This makes it accessible to non-programmers but raises concerns about reliability and debugging.
1.2 How Vibe Coding Differs from Other Methods
In traditional coding, developers manually write and debug every line of code, requiring extensive technical knowledge. Low-code platforms simplify this process by offering drag-and-drop tools but still require some understanding of programming concepts. Vibe coding takes this a step further by allowing users to communicate their requirements in natural language, making it highly accessible even to those without any programming background. However, it is less precise and reliable for complex projects compared to manual coding.
1.3 Why is Security Crucial in Vibe Coding?
AI-generated code isn't inherently secure. It can miss critical security best practices, leaving your applications vulnerable to attacks. This guide is designed to help you bridge that gap, ensuring that your vibe-coded projects are not only innovative but also secure.
For example, research has shown that LLM-generated code is inherently insecure, with vulnerabilities often slipping through standard evaluations. To address this, BaxBench—a benchmark developed by LogicStar (an AI startup) and researchers at ETH Zurich—tests LLMs’ ability to produce secure backend code. BaxBench evaluates 392 security-critical tasks across 6 languages and 14 frameworks, combining functional correctness checks with expert-designed security exploits. This standardized, community-driven framework aims to improve code safety by exposing flaws in LLM outputs and guiding future model enhancements. See the figure below, the top foundational models generate at least 36% of insecure code.
2: The Secure Vibe Coding Checklist
This checklist covers essential security practices across the entire development lifecycle. We've broken it down into key categories to make it easy to understand and implement.
2.1 Vibe Coding Security Fundamentals
Let's start with the fundamentals of secure vibe coding. These are the principles to keep in mind as you're generating code with AI:
- Avoid Hardcoding Sensitive Data: Never embed API keys, secrets, database passwords, or other sensitive information directly in your code. Instead, use environment variables or a secure secrets management system.
- Tip: When prompting AI to generate code, explicitly request the use of environment variables for sensitive configuration.
- Secure API Endpoints: Always implement robust authentication (e.g., OAuth) and authorization mechanisms for all your API endpoints. Ensure that only authorized users can access sensitive data or functionality.
- Tip: Use AI to help you generate secure endpoint configurations, including access control lists and authentication policies.
- Validate Inputs: Sanitize and validate all user inputs to prevent injection attacks like SQL injection, cross-site scripting (XSS), and command injection. Use appropriate libraries and techniques to escape user-provided data.
- Tip: Carefully review AI-generated code to ensure it includes proper input validation and sanitization routines.
- Configure CORS Properly: Cross-Origin Resource Sharing (CORS) controls which domains are allowed to access your application's resources. Configure CORS carefully, restricting access to only trusted domains. Avoid using wildcard (*) settings, as they can open your application to unauthorized access.
- Tip: Double-check the CORS settings generated by AI tools to ensure they are restrictive and secure.
- Use HTTPS: Always use HTTPS for your web projects to encrypt data in transit and protect against eavesdropping. Ensure that your server is properly configured with a valid SSL/TLS certificate.
- Tip: Verify that AI-generated web configurations include HTTPS and enforce its use.
- Regular Code Reviews: Conduct regular code reviews to identify and address potential security vulnerabilities. Use AI-powered code analysis tools like Grok to assist in the review process, but also involve human reviewers, especially for critical projects and sensitive areas of your codebase.
- Tip: When using AI code review tools, prompt them with specific security-related queries, such as "Check for security mistakes" or "Identify potential injection vulnerabilities."
- Educate on Security Basics: Understand fundamental security principles like the principle of least privilege, separation of concerns, and defense in depth. This knowledge will help you identify and mitigate potential vulnerabilities in your code.
- Tip: Utilize resources like the OWASP (Open Web Application Security Project) website to learn more about secure coding practices.
- Seek Peer Review: Have experienced developers review your code to catch overlooked issues and provide feedback on your security practices. Leverage collaborative platforms like GitHub for peer reviews and security assessments.
- Tip: Encourage open communication and knowledge sharing within your development team regarding security best practices.
2.2 Application Security (AppSec): Building a Solid Foundation
AppSec is about integrating security into every stage of your software development process. We'll use guidelines from the OWASP Secure Coding Practices Quick Reference Guide to ensure a robust foundation:
- Least Privilege: Grant users only the necessary permissions to perform their tasks. Don't give everyone admin access!
- Data Encryption: Encrypt sensitive data both in transit and at rest. Use strong encryption algorithms like AES-256.
- CI/CD Security Scanning: Integrate security scanning tools like Snyk and Checkmarx into your CI/CD pipeline to automatically detect vulnerabilities. Use Invicti for dynamic testing.
- Regular Security Audits: Conduct regular security audits, including penetration testing, to identify and address potential weaknesses.
- Remove console.log() Statements: Debugging is essential, but those console.log() statements can expose sensitive data in production. Use a build process to automatically remove them.
- Hide System Errors from the User: Don't display detailed error messages to end-users. Instead, show user-friendly messages and log the detailed errors for debugging.
2.3. API Security: Protecting Your Endpoints
APIs are a frequent target for attackers. Follow these guidelines based on OWASP's API Security Top 10 to secure your endpoints:
- Use HTTPS: Encrypt API communications to protect data in transit. Ensure TLS 1.2 or higher is used.
- Authentication and Authorization: Use OAuth, JWT, or API keys for secure access control. Implement token expiration and refresh mechanisms.
- Input Validation: Sanitize inputs to prevent injection attacks, such as SQL or XSS. Use libraries like Express Validator for Node.js.
- Rate Limiting and Throttling: Limit requests to prevent abuse, such as DDoS attacks. Configure limits based on expected traffic.
- Use API Gateways: Leverage gateways for logging, monitoring, and additional security layers. Consider AWS API Gateway or similar.
- Implementing Rate Limiting: Use tools like Redis or Next.js Middleware to prevent denial-of-service (DoS) attacks and brute-force attempts. Start with conservative limits and adjust based on monitoring.
2.4 GitHub Security: Securing Your Code Repository
Your GitHub repository is a goldmine for attackers if it's not properly secured. Here's how to protect it:
- Enable Two-Factor Authentication (2FA): This is a must-have for all developers.
- Keep Repositories Private: Sensitive projects should always be kept private to prevent public exposure.
- Use Dependabot: Automatically update dependencies and receive alerts on known vulnerabilities.
- Secure Webhooks and API Tokens: Store these securely using GitHub Secrets.
- Regularly Review Access Permissions: Ensure only authorized users have access to the repository.
- Avoid Pushing .env files or Hardcoding API Keys: Never commit .env files containing sensitive information. Use environment variables and GitHub Secrets.
2.5 Database Security: Protecting Your Data
Databases are a prime target for attackers. Use these guidelines based on OWASP's Database Security Cheat Sheet to keep your data safe:
- Parameterized Queries: Use prepared statements to prevent SQL injection. Always validate inputs before querying.
- Data Encryption: Encrypt sensitive data at rest and in transit, using AES-256. Use cloud provider KMS for key management.
- Least Privilege Access: Limit database permissions to necessary operations only. Regularly audit and revoke unused permissions.
- Regular Backups: Ensure secure backups and test restore procedures. Encrypt backups to prevent data exposure.
- Monitor Activity: Use logging and monitoring tools to detect unusual patterns. Set alerts for suspicious activity, like mass deletes.
- Frontend to Database: Prevent the frontend directly talk to your database. Use API routes (server-side functions) to fetch data securely.
2.6 OWASP LLM Top 10: Addressing AI-Specific Risks
If you're building applications that leverage Large Language Models (LLMs), you need to be aware of these emerging risks:
- LLM01:2025 Prompt Injection: User prompts can alter the LLM's behavior in unintended ways. Mitigate by constraining model behavior, validating output formats, and implementing input/output filtering.
- LLM02:2025 Sensitive Information Disclosure: LLMs can expose sensitive data through their output. Mitigate by integrating data sanitization, robust input validation, and strict access controls.
- LLM03:2025 Supply Chain: LLM supply chains are vulnerable to risks affecting training data, models, and deployment platforms. Mitigate by vetting data sources, applying vulnerability scanning, and maintaining an up-to-date inventory.
- LLM04:2025 Data and Model Poisoning: Training data can be manipulated to introduce vulnerabilities. Mitigate by tracking data origins, validating model outputs, and implementing sandboxing and anomaly detection.
- LLM05:2025 Improper Output Handling: Insufficient validation of LLM outputs can lead to vulnerabilities. Mitigate by adopting a zero-trust approach, following OWASP ASVS guidelines for input validation, and encoding outputs.
- LLM06:2025 Excessive Agency: LLM-based systems granted too much autonomy can perform damaging actions. Mitigate by minimizing extensions, limiting permissions, and requiring human approval for high-impact actions.
- LLM07:2025 System Prompt Leakage: System prompts can expose sensitive information. Mitigate by separating sensitive data from system prompts and enforcing security controls independently of the LLM.
- LLM08:2025 Vector and Embedding Weaknesses: Vulnerabilities in vectors and embeddings can be exploited to inject harmful content. Mitigate by implementing fine-grained access controls and validating data sources.
- LLM09:2025 Misinformation: LLMs can produce false or misleading information. Mitigate by using Retrieval-Augmented Generation, encouraging human oversight, and implementing automatic validation mechanisms.
- LLM10:2025 Unbounded Consumption: LLMs can allow excessive, uncontrolled inferences, risking denial of service. Mitigate by implementing strict input validation, rate limiting, and dynamic resource allocation.
2.7. Cloud Deployment Security (Example: Vercel)
Vercel is a popular platform used by vibe coders to deploy web applications built by v0 tool. Take advantage of these security features:
- Vercel Firewall and DDoS Mitigation: Leverage Vercel's built-in protection against malicious traffic.
- HTTPS and SSL Certificates: Vercel automatically handles HTTPS and SSL certificates for secure communication.
- Access Control: Use password protection and Single Sign-On (SSO) to control access to your deployments.
- Logs and Source Protection: Secure your build logs and source code to prevent unauthorized access.
- Follow Vercel's Production Checklist: Ensure your application meets operational excellence, security, reliability, performance, and cost optimization standards.
- Sanitize User Inputs: Sanitize user inputs to prevent code execution. Use server-side validation and encoding. Use libraries like DOMPurify or framework-specific sanitization functions.
- Secure Login System: Use well-established authentication providers like Auth0, Firebase Authentication, or Vercel Auth instead of building your own login system from scratch.
- Configure Correctly Environment Variables: Ensure environment variables are correctly set for production and not exposed in the client-side code. Use platforms like Vercel to manage environment variables securely.
2.8. The Human Factor: Expertise and Continuous Learning
- Hire a Professional: If you're not confident in your security expertise, consider hiring a security expert or a developer with strong security knowledge.
- Consult Security Experts: If you are unsure, consult with a security expert or hire a developer with experience in secure coding practices and conduct code reviews with a security focus.
- Stay Informed: The security landscape is constantly evolving. Stay up-to-date with the latest vulnerabilities and best practices.
3. Secure Vibe Coding Prompts
This section provides suggested prompts to use with Code Agent to incorporate security considerations into your vibe coding workflow. Use these prompts as starting points and adapt them to your specific needs.
3.1 Vibe Coding Security Fundamentals Prompts
- Avoid Hardcoding Sensitive Data:
- "Generate code to connect to a database using environment variables for the database URL, username, and password."
- "Create an API endpoint that authenticates using an API key stored securely in an environment variable."
- Secure API Endpoints:
- "Generate an Express.js API endpoint that requires authentication using JWT (JSON Web Token) and authorization based on user roles."
- "Create a function that checks if a user has the necessary permissions to access a specific resource before granting access."
- Validate Inputs:
- "Generate a function that sanitizes user input to prevent XSS (Cross-Site Scripting) attacks."
- "Create a function that validates user input to ensure it matches a specific format or range before processing it."
- Configure CORS Properly:
- "Generate a middleware function that configures CORS to only allow requests from a specific domain."
- "Create a configuration that sets the CORS headers to allow requests from 'https://example.com'."
- Use HTTPS:
- "Generate a server configuration that redirects all HTTP requests to HTTPS."
- "Create a script that automatically renews SSL certificates using Let's Encrypt."
- Regular Code Reviews:
- "Analyze the following code for potential security vulnerabilities, including injection attacks, authentication bypasses, and data breaches."
- "Identify areas in the codebase where security best practices are not being followed."
- Educate on Security Basics:
- "Explain the principle of least privilege and how it applies to web application security."
- "Describe the common types of web application vulnerabilities and how to prevent them."
- Seek Peer Review:
- "Suggest security-focused questions to ask during a code review of a web application."
- "Create a checklist of security considerations for reviewing API endpoints."
3.2 Application Security (AppSec) Prompts
- Least Privilege:
- "Generate code that implements role-based access control (RBAC) in a web application, ensuring users only have access to the features they need."
- Data Encryption:
- "Generate code that encrypts sensitive data in transit using HTTPS and at rest using AES-256 encryption."
- CI/CD Security Scanning:
- "Give me some best prompts to test security vulnerabilities such as Snyk and Checkmarx into your CI/CD pipeline."
- Regular Security Audits:
- "Give me the prompts for the penetration testing and identifying the vulnerabilities."
- Remove console.log() Statements:
- "Generate a script that automatically removes all console.log() statements from a JavaScript codebase during the build process."
- Hide System Errors from the User:
- "Generate code that catches exceptions and displays user-friendly error messages instead of exposing internal system details."
3.3 API Security Prompts
- Use HTTPS:
- "Generate code that enforces HTTPS for all API requests."
- Authentication and Authorization:
- "Generate an API endpoint that requires authentication using JWT and authorization based on user roles."
- Input Validation:
- "Generate a function that sanitizes user input to prevent SQL injection attacks."
- Rate Limiting and Throttling:
- "Generate a middleware function that limits the number of requests from a single IP address to prevent DDoS attacks."
- Use API Gateways:
- "Give me the prompts to implement API gateways for the logging, monitoring and any extra security layer."
3.4 GitHub Security Prompts
- Enable Two-Factor Authentication (2FA):
- "Explain how to enable two-factor authentication on a GitHub account."
- Keep Repositories Private:
- "Give me the steps or prompts for keeping the repositories private."
- Use Dependabot:
- "Explain how to configure Dependabot to automatically update dependencies and receive alerts on vulnerabilities."
- Secure Webhooks and API Tokens:
- "Generate code that encrypts API tokens before storing them in a database."
- Regularly Review Access Permissions:
- "Give me prompts for doing permission reviews."
- Avoid Pushing .env Files or Hardcoding API Keys:
- " Explain how and why avoid pushing .env files to the github."
3.5 Database Security Prompts
- Parameterized Queries:
- "Generate code that uses parameterized queries to prevent SQL injection attacks."
- Data Encryption:
- "Generate code that encrypts sensitive data at rest using AES-256 encryption."
- Least Privilege Access:
- "Give me prompts for giving or limiting the database permission"
- Regular Backups:
- "Give me prompts to ensure secure database backups"
- Monitor Activity:
- "Give me prompts for logging any suspicious activity from database."
- Frontend to Database:
- "Explain in the prompts to limit the front end to directly interact with databases."
3.6 OWASP LLM Top 10 Prompts
- LLM01:2025 Prompt Injection:
- "Generate a system prompt that prevents users from altering the LLM's behavior or output in unintended ways."
- "Create code that validates the format and content of LLM outputs to ensure they adhere to predefined rules."
- LLM02:2025 Sensitive Information Disclosure:
- "Generate a script that sanitizes LLM training data to remove or mask sensitive information."
- "Create a function that filters user input to prevent the injection of sensitive data into LLM prompts."
- LLM03:2025 Supply Chain:
- "Generate code that verifies the integrity of LLM models and datasets before using them in an application."
- LLM04:2025 Data and Model Poisoning:
- "Generate a system that detects and mitigates data poisoning attacks on LLM training data."
- LLM05:2025 Improper Output Handling:
- "Generate code that validates and sanitizes LLM outputs before using them in other systems to prevent XSS and other attacks."
- LLM06:2025 Excessive Agency:
- "Generate code that limits the functionality and permissions of LLM-based systems to prevent them from performing damaging actions."
- LLM07:2025 System Prompt Leakage:
- "Generate a system prompt that does not expose sensitive information or instructions about the LLM's internal workings."
- LLM08:2025 Vector and Embedding Weaknesses:
- "Generate code that implements fine-grained access controls for vector stores to prevent unauthorized access to sensitive data."
- LLM09:2025 Misinformation:
- "Generate code that uses Retrieval-Augmented Generation (RAG) to enhance the reliability of LLM outputs with verified data."
- LLM10:2025 Unbounded Consumption:
- "Generate code that implements rate limiting and user quotas to prevent LLMs from consuming excessive resources."
3.7 Cloud Deployment Security (Focus on Vercel) Prompts
- Vercel Firewall and DDoS Mitigation:
- "Give prompts to how to leverage the Vercel to prevent from DDOS attacks and other attacks"
- HTTPS and SSL Certificates:
- "How vercel manages HTTPS and SSL certfications?"
- Access Control:
- "What are the prompts for using or setting up the access control."
- Logs and Source Protection:
- "How do i enable logs and source protection in vercel."
- Follow Vercel's Production Checklist:
- "Give me example to follow Vercel's production checlist."
- Sanitize User Inputs:
- "Generate a function that sanitizes user input to prevent code execution on the server."
- Secure Login System:
- "Generate code that integrates with Auth0 for secure user authentication."
- Configure Correctly Environment Variables:
- "Explain how to securely configure environment variables in Vercel."
3.8 The Human Factor Prompts
- Hire a Professional:
- "Where can I find qualified security professionals or developers with strong security expertise?"
- "I want prompts to find security professionals in my domain."
- Consult Security Experts:
- "I want prompts to consult from security professional and a developer."
- Stay Informed:
- "Recommend resources for staying up-to-date on the latest security vulnerabilities and best practices."
- "Suggest security blogs, newsletters, and conferences to follow."
Remember to adapt these prompts to your specific needs and context. Happy and secure vibe coding!
4: Conclusion: Secure Vibe Coding is a Shared Responsibility
Vibe coding is transforming software development, but it's crucial to prioritize security from the start. By following this comprehensive guide and staying vigilant, you can build secure, reliable, and innovative applications that are ready for the real world. Remember, security is not a one-time fix; it's an ongoing process. Happy (and secure!) vibe coding!
About the Author
Ken Huang is a prolific author and renowned expert in AI and Web3, with numerous published books spanning AI and Web3 business and technical guides and cutting-edge research. As Co-Chair of the AI Safety Working Groups at the Cloud Security Alliance, and Co-Chair of AI STR Working Group at World Digital Technology Academy under UN Framework, he's at the forefront of shaping AI governance and security standards.
Huang also serves as CEO and Chief AI Officer(CAIO) of DistributedApps.ai, specializing in Generative AI related training and consulting. His expertise is further showcased in his role as a core contributor to OWASP's Top 10 Risks for LLM Applications and his active involvement in the NIST Generative AI Public Working Group in the past.
Key Books
- “Agentic AI: Theories and Practices” (upcoming, Springer, August, 2025)
- "Beyond AI: ChatGPT, Web3, and the Business Landscape of Tomorrow" (Springer, 2023) - Strategic insights on AI and Web3's business impact.
- "Generative AI Security: Theories and Practices" (Springer, 2024) - A comprehensive guide on securing generative AI systems
- "Practical Guide for AI Engineers" (Volumes 1 and 2 by DistributedApps.ai, 2024) - Essential resources for AI and ML Engineers
- "The Handbook for Chief AI Officers: Leading the AI Revolution in Business" (DistributedApps.ai, 2024) - Practical guide for CAIO in small or big organizations.
- "Web3: Blockchain, the New Economy, and the Self-Sovereign Internet" (Cambridge University Press, 2024) - Examining the convergence of AI, blockchain, IoT, and emerging technologies
His co-authored book on "Blockchain and Web3: Building the Cryptocurrency, Privacy, and Security Foundations of the Metaverse" (Wiley, 2023) has been recognized as a must-read by TechTarget in both 2023 and 2024.
A globally sought-after speaker, Ken has presented at prestigious events including Davos WEF, ACM, IEEE, CSA AI Summit, IEEE, ACM, Depository Trust & Clearing Corporation, and World Bank conferences.
Ken Huang is a member of OpenAI Forum to help advance its mission to foster collaboration and discussion among domain experts and students regarding the development and implications of AI.
Related Resources
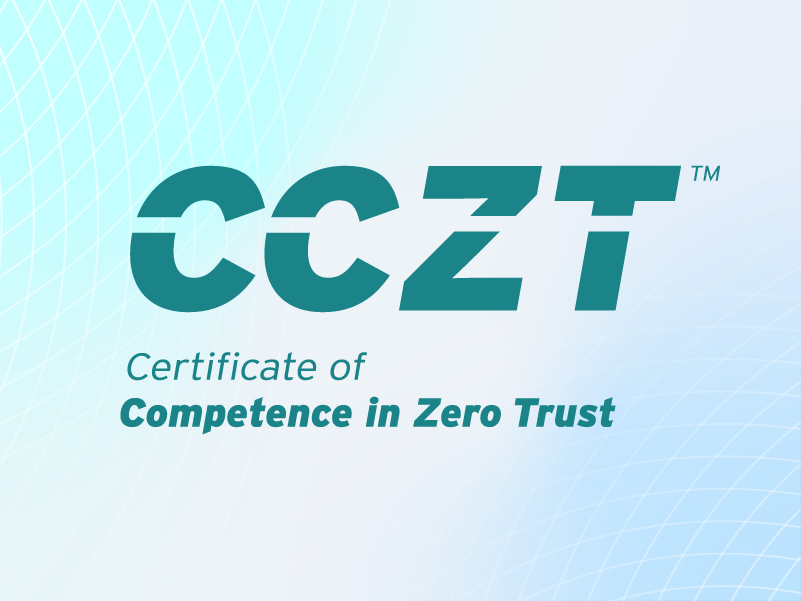
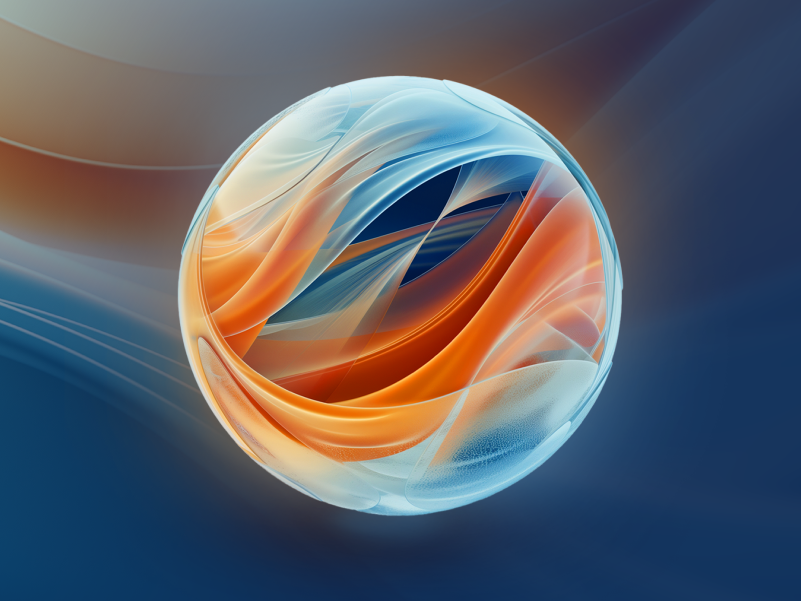
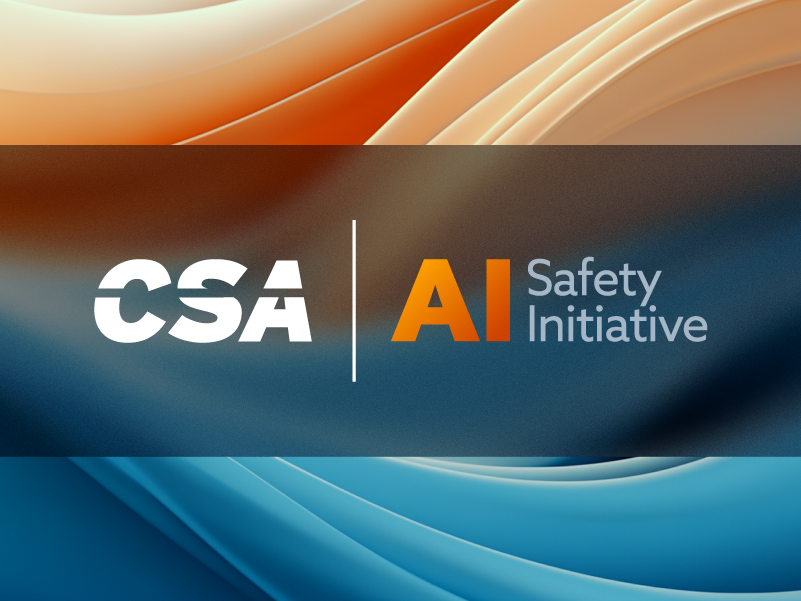
Unlock Cloud Security Insights
Subscribe to our newsletter for the latest expert trends and updates
Related Articles:
A Copilot Studio Story: Discovery Phase in AI Agents
Published: 06/26/2025
What Kind of Identity Should Your AI Agent Have?
Published: 06/25/2025
A Primer on Model Context Protocol (MCP) Secure Implementation
Published: 06/23/2025
Cloud Security: Whose Job Is It?
Published: 06/23/2025